Code updates
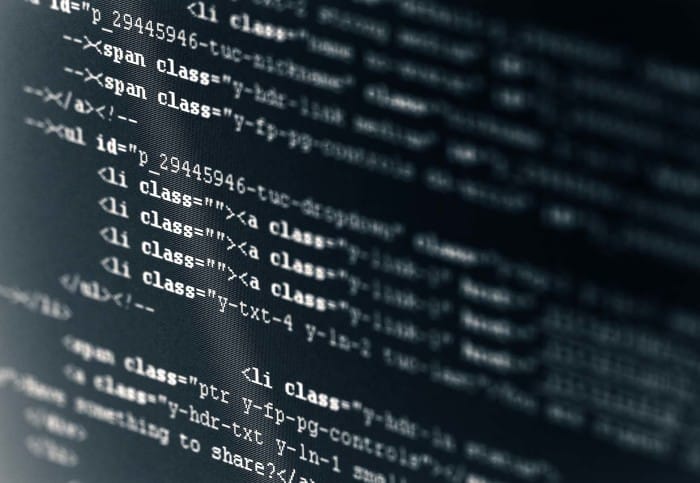
I have taken some time to update my GitHub repository, who has been lonely as of date. While developing code for my projects at the university, I came to realise that much of this code could prove useful to others.
CSPopViewService
First thing I did was to update the pop service for iOS library. The library is constructed to work with Chris Miles’ excellent CMPopTipView library, and improve automatisation of the appearance and disappearance of the pop tip views. The need for this came from the time I needed to construct a live demo with functionality from the application, so we needed to present and dismiss pop tip views automatically and at arbitrary time intervals.
Consider this code, taken from CSPopViewService.h
/** Presents the view after a delay @param view the popTipView to display @param delay after how many seconds will it be presented? @param barButtonItem the bar button item to point the view at @param dismissOther dismiss other views while presenting this one? */ - (void) presentPopView:(CMPopTipView *)view afterDelay:(NSTimeInterval)delay pointingAtBarButtonItem:(UIBarButtonItem *)barButtonItem dismissOtherManagedPopViews:(BOOL)dismissOther;
And here is how it is actually used:
CMPopTipView *pop = [[CMPopTipView alloc] initWithTitle:@"Title" message:@"Message"]; CMPopTipView *pop2 = [[CMPopTipView alloc] initWithTitle:@"Title" message:@"Message"]; CMPopTipView *pop3 = [[CMPopTipView alloc] initWithTitle:@"Title" message:@"Message"]; self.popService = [[CSPopViewService alloc] init]; [self.popService presentPopView:pop afterDelay:2 pointingAtBarButtonItem:self.barItem1 dismissOtherManagedPopViews:NO]; [self.popService presentPopView:pop2 afterDelay:3 pointingAtBarButtonItem:self.barItem2 dismissOtherManagedPopViews:NO]; [self.popService presentPopView:pop3 afterDelay:5 pointingAtBarButtonItem:self.barItem3 dismissOtherManagedPopViews:YES];
This will have the following effect:
- It will present the ‘pop’ object pointing at the first bar item 2 seconds after the command is given.
- It will present the ‘pop2’ object pointing at the second bar item 1 second after the ‘pop’ item will appear.
- It will present the ‘pop3’ object pointing at the second bar item 2 seconds after the ‘pop2’ item appears. The other 2 pop items will be dismissed when ‘pop3’ appears.
That way, you can make presentation or tutorials within your application. You will not need to worry about threading or timers, since all timers can be cleaned up using the -stopAllTimers function. The best place for this function is the -viewWillDisappear function inside your view controller.
Sequential Reader – seqreader
Sequential Reader is a (little for the time being) file reader that I made, aimed at facilitating parsing files. I wanted a system that is fast and memory efficient, and can parse a file line by line. I had some line-by-line parsing to do in a HUGE file, to complete a project for the university. Turns out that many people had the same request as me, as Apple did not provide an easy way to do that.
Anyway, CSFileReader was created for this purpose. Just instantiate the reader with the file path, its encoding, and then assign a delegate to it. While parsing, the file will send you callbacks through the delegate functions, with the line that was read in NSString format.
—
I will have to invest some more time to these libraries to make them more mature. I will also have to create a new repository for CSWatchDog, a nice simple wrapper around GCD timers, aimed at facilitating working with events about to be fired after a few seconds. More on this, and updated code, soon.