AFNetworking + PromiseKit
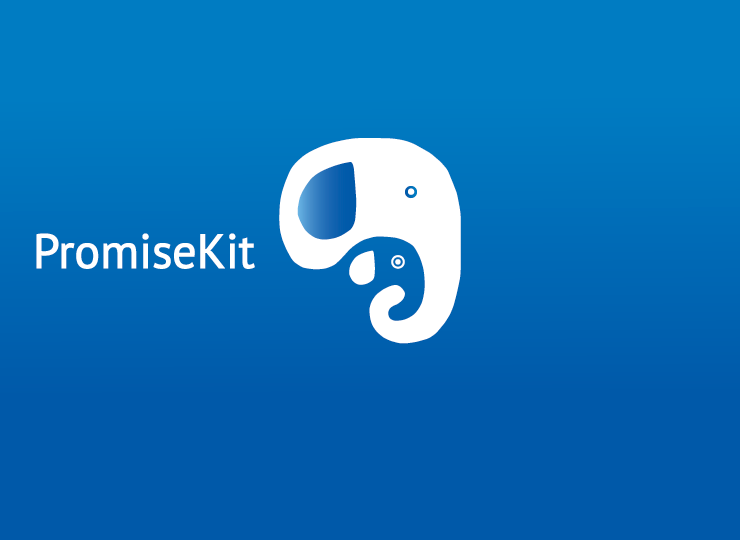
AFNetworking is the de-facto standard for implementing a robust and scalable network infrastructure in your iOS or OS X application. I personally have used it for the implementation of many professional projects that I have been involved in. And lately I have stumbled across an delightful Framework, PromiseKit, a Promises implementation for iOS. I have then taken the liberty of incorporating Promises features into AFNetworking.
PromiseKit + AFNetworking is a category on AFNetworking, covering AFHTTPRequestOperation, AFHTTPRequestOperationManager and AFHTTPSessionManager .
An example use of PromiseKit + AFNetworking:
[AFHTTPRequestOperation request:[NSURLRequest requestWithURL:[NSURL URLWithString:@"http://oramind.com/"]]].then(^(id responseObject){ NSLog(@"operation completed! %@", [[NSString alloc] initWithData:responseObject encoding:NSUTF8StringEncoding]); }).catch(^(NSError *error){ NSLog(@"error: %@", error.localizedDescription); NSLog(@"original operation: %@", error.userInfo[AFHTTPRequestOperationErrorKey]); }); self.manager = [[AFHTTPRequestOperationManager alloc] initWithBaseURL:nil]; self.manager.responseSerializer = [AFHTTPResponseSerializer serializer]; [self.manager GET:@"http://www.oramind.com/" parameters:nil].then(^(id responseObject, AFHTTPRequestOperation *operation){ NSLog(@"first request completed for operation: %@", operation.request); return [self.manager GET:@"http://www.apple.com" parameters:nil]; }).then(^{ NSLog(@"second request completed"); }).catch(^(NSError *error){ NSLog(@"error happened: %@", error.localizedDescription); NSLog(@"original operation: %@", error.userInfo[AFHTTPRequestOperationErrorKey]); });
Sample function that logins using an AFHTTPSessionManager below:
- (PMKPromise *)loginWithUserName:(NSString *)userName andPassword:(NSString *)password { return [self.sessionManager POST:@"api/login" parameters:@{@"user": userName, @"password" : password}].then(^(id responseObject, NSURLSessionDataTask *dataTask){ //responseObject holds the response returned by AFNetworking //dataDask will hold an NSURLSessionDataTask associated with this request, like it happens in AFHTTPSessionManager }); }
In order to include these capabilities to your project, you have two options: Either use cocoapods (look into the appropriate section below), or ust copy AFNetworking+Promises.h and AFNetworking+Promises.m in your project and use them.
Source code, and detailed README: https://github.com/csotiriou/AFNetworking-PromiseKit
PromiseKit: http://promisekit.org
AFNetworking: http://github.com/AFNetworking/AFNetworking